The Dining Philosophers Problem using java
- Pankajan Kajan
- Apr 3, 2019
- 3 min read
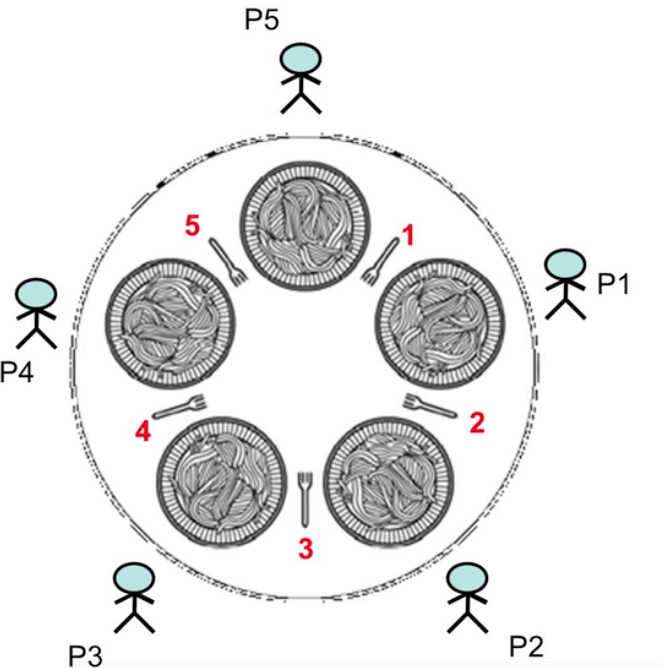
The Dining Philosophers problem is the very famous problem that describe synchronization issues in a multi-threaded environment and illustrate techniques for solving them. Dijkstra first formulated this problem and presented it regarding computers accessing tape drive peripherals.
The present formulation was given by Tony Hoare, who is also known for inventing the quicksort sorting algorithm. In this article, we analyze this well-known problem and code a popular solution.
The diagram above represents the problem. There are five silent philosophers (P1 – P5) sitting around a circular table, spending their lives eating and thinking. they only do that jobs.
There are five forks for them to share (1 – 5) and to be able to eat, a philosopher needs to have forks in both his hands. After eating, he puts both of them down and then they can be picked by another philosopher who repeats the same cycle. We want to show this how we can solve this problem.
I use java language to solve this problem. Java we can use concurrency easily. I am familiar with the java language. Here We can face the dead lock also when we start programming we must consider the dead lock also. Each of the Philosophers has acquired his left fork, but can’t acquire his right fork, because his neighbor has already acquired it. This situation is commonly known as the circular wait and is one of the conditions that results in a deadlock and prevents the progress of the system. So we must consider this situation also.
First I create the needed objects food, fork, philosopher these are my objects that i use in my program.
In the food object that have the amount of food that decide when the philosophers stop the loop.
public class Food { private int food; public Food(int food) { this.food = food; } public int getFood() { return food; } public void setFood(int food) { this.food -= food; } }
and I create the fork that the philosophers use to eat the food. It have the fork number that start from the 0-4. it is use to identify the fork.
package com.company; public class Fork { private int no; public Fork(int no) { this.no = no; } public int getNo() { return no; } }
After that i create the philosopher class that use the concurrency to eat the food. when the philosopher get two spoons then he start to eat otherwise he do only thinking. The eating and the thinking time is decided by the random number generator. It generate 0-100; using that it will decide the time of the job that philosophers do. we use the synchronized keyword to acquire the internal monitor of the fork object and prevent other threads from doing the same.
public class Philosopher implements Runnable{ //The forks that use by philosophers private Fork leftFork,rightFork; private Food food=null; private int amount; public Philosopher(Fork leftFork, Fork rightFork, int food) { this.leftFork = leftFork; this.rightFork = rightFork; this.food = new Food(food); } //do the action that eating or thinking private void perform(String action){ System.out.printf("%s %s\n",Thread.currentThread().getName(),action); amount = ((int) (Math.random() * 100)); try { Thread.sleep(amount); } catch (InterruptedException e) { e.printStackTrace(); } } @Override public void run() { while(food.getFood() > 0){ //start from philosophers thinking perform("Thinking"); synchronized (leftFork) { perform(" Picked up left fork "+leftFork.getNo()); synchronized (rightFork) { // eating perform("Picked up right fork "+rightFork.getNo()+" - eating"); food.setFood(amount); perform("eat:"+amount+" .balance:"+food.getFood()+" . Put down right fork "+rightFork.getNo()); } // Back to thinking perform(" Put down left fork. Back to thinking"); } } } }
In the main method I create the two arrays that contain the philosophers object and the fork object. we create food and the philosopher object . when we create the philosopher object I give two fork (left and right) and the amount of the food.
public class Main { public static void main(String[] args) { int number = 5; //create the object holders Philosopher philosophers[] = new Philosopher[number]; Fork forks[] = new Fork[number]; for(int c = 0; c<number; c++) { forks[c] = new Fork(c); } for (int i = 0; i < number; i++) { Fork leftFork = forks[i]; Fork rightFork = forks[(i + 1) % number]; if (i == number - 1) { // The last philosopher picks up the right fork first philosophers[i] = new Philosopher(rightFork, leftFork, 200); } else { philosophers[i] = new Philosopher(leftFork, rightFork, 200); } Thread t = new Thread(philosophers[i], "Philosopher " + (i + 1)); t.start(); } } }
This is the solution to the Dinning philosopher problem. If you want to access the code so you can click this link: https://github.com/pankajan05/Dinning-Philosopher-Problem-
Comments